zensols.dsprov package#
Submodules#
zensols.dsprov.app#
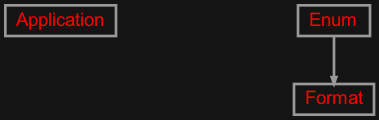
This library provides integrated MIMIC-III with discharge summary provenance of data annotations and Pythonic classes.
- class zensols.dsprov.app.Application(stash)[source]#
Bases:
object
This library provides integrated MIMIC-III with discharge summary provenance of data annotations and Pythonic classes.
- __init__(stash)#
- admissions(limit=None)[source]#
Print the annotated admission IDs.
- Parameters:
limit (
int
) – the limit on items to print
-
stash:
Stash
# A stash that creates
AdmissionMatch
instances.
zensols.dsprov.cli#
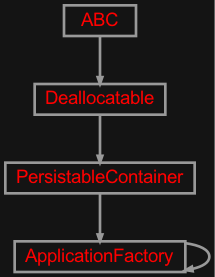
Command line entry point to the application.
- class zensols.dsprov.cli.ApplicationFactory(*args, **kwargs)[source]#
Bases:
ApplicationFactory
zensols.dsprov.domain#
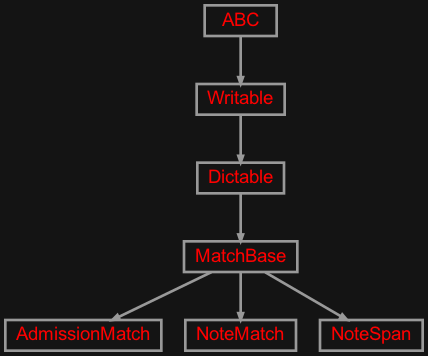
Container classes for discharge summary to note antecedent match data.
- class zensols.dsprov.domain.AdmissionMatch(note_matches)[source]#
Bases:
MatchBase
Contains match data for an admission.
- __init__(note_matches)#
- write(depth=0, writer=<_io.TextIOWrapper name='<stdout>' mode='w' encoding='utf-8'>)[source]#
Write this instance as either a
Writable
or as aDictable
. If class attribute_DICTABLE_WRITABLE_DESCENDANTS
is set asTrue
, then use thewrite()
method on children instead of writing the generated dictionary. Otherwise, write this instance by first creating adict
recursively usingasdict()
, then formatting the output.If the attribute
_DICTABLE_WRITE_EXCLUDES
is set, those attributes are removed from what is written in thewrite()
method.Note that this attribute will need to be set in all descendants in the instance hierarchy since writing the object instance graph is done recursively.
- Parameters:
depth (
int
) – the starting indentation depthwriter (
TextIOBase
) – the writer to dump the content of this writable
- class zensols.dsprov.domain.MatchBase[source]#
Bases:
Dictable
A base class for match data containers that enforces no pickling/serialization of note spans. This is not supported as subclasses contain complex object graphs.
- __init__()#
- class zensols.dsprov.domain.NoteMatch(hadm_id, discharge_summary, antecedents, source)[source]#
Bases:
MatchBase
A match between a text span in the discharge summary with the semanically similar or copy/pasted text with the note antecedents. This is the analog to the
MatchedAnnotation
in the reproducibility repo.- __init__(hadm_id, discharge_summary, antecedents, source)#
- write(depth=0, writer=<_io.TextIOWrapper name='<stdout>' mode='w' encoding='utf-8'>, include_sections=False)[source]#
Write this instance as either a
Writable
or as aDictable
. If class attribute_DICTABLE_WRITABLE_DESCENDANTS
is set asTrue
, then use thewrite()
method on children instead of writing the generated dictionary. Otherwise, write this instance by first creating adict
recursively usingasdict()
, then formatting the output.If the attribute
_DICTABLE_WRITE_EXCLUDES
is set, those attributes are removed from what is written in thewrite()
method.Note that this attribute will need to be set in all descendants in the instance hierarchy since writing the object instance graph is done recursively.
- Parameters:
depth (
int
) – the starting indentation depthwriter (
TextIOBase
) – the writer to dump the content of this writable
- class zensols.dsprov.domain.NoteSpan(lexspan, note)[source]#
Bases:
MatchBase
A tie between two spans of semantically similar or copied text segments between a note antecedent and a discharge summary This is the analog to
MatchedNote
in the reproducibility repo, but use paper terminology.- __init__(lexspan, note)#
-
lexspan:
LexicalSpan
# The 0-index start and end offset in
note
the demarcates the span lexically.
- property span: TokenContainer#
The span as features demarcated by the span.
- write(depth=0, writer=<_io.TextIOWrapper name='<stdout>' mode='w' encoding='utf-8'>, include_sections=False)[source]#
Write this instance as either a
Writable
or as aDictable
. If class attribute_DICTABLE_WRITABLE_DESCENDANTS
is set asTrue
, then use thewrite()
method on children instead of writing the generated dictionary. Otherwise, write this instance by first creating adict
recursively usingasdict()
, then formatting the output.If the attribute
_DICTABLE_WRITE_EXCLUDES
is set, those attributes are removed from what is written in thewrite()
method.Note that this attribute will need to be set in all descendants in the instance hierarchy since writing the object instance graph is done recursively.
- Parameters:
depth (
int
) – the starting indentation depthwriter (
TextIOBase
) – the writer to dump the content of this writable
zensols.dsprov.stash#
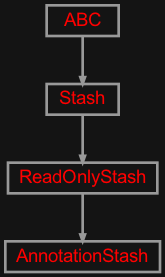
A stash class used for accessing the provenance of data annotations.
- class zensols.dsprov.stash.AnnotationStash(installer, corpus)[source]#
Bases:
ReadOnlyStash
A stash that create instances of
AdmissionMatch
.- __init__(installer, corpus)#
- exists(hadm_id)[source]#
Return
True
if data with keyname
exists.Implementation note: This
Stash.exists()
method is very inefficient and should be overriden.- Return type:
-
installer:
Installer
#